Curl in Python
In this article, we'll explore how to use the curl library in Python to make HTTP requests and handle responses.

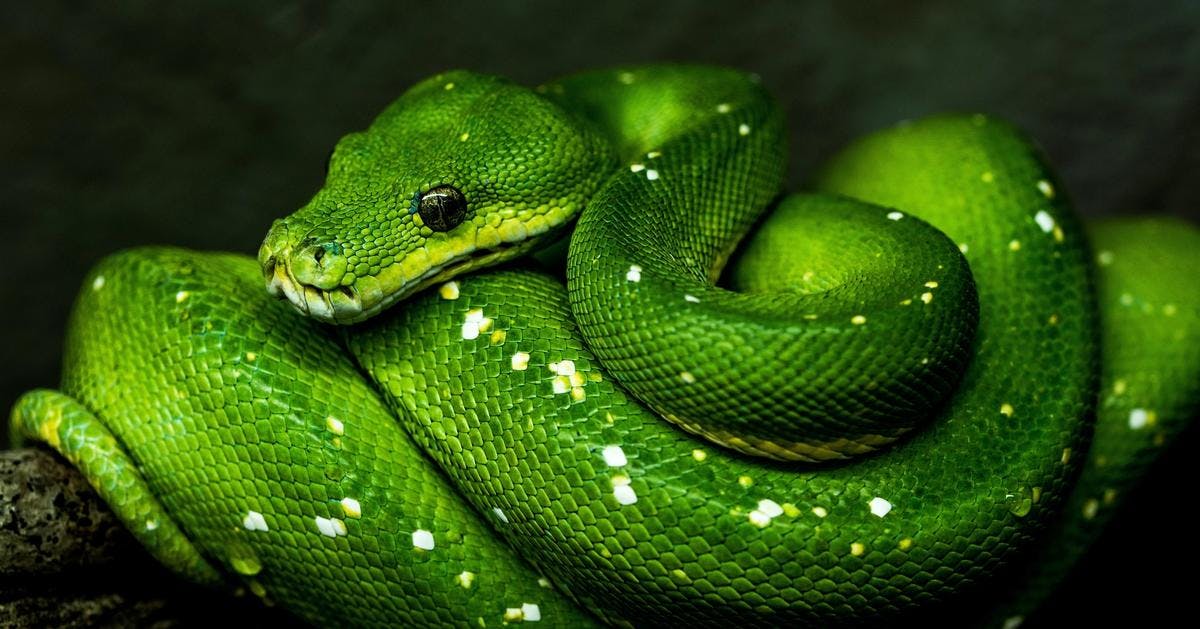
Python is a popular programming language that is widely used for a variety of purposes, including web development, data analysis, and automation. One of the reasons for its popularity is the extensive collection of libraries and frameworks that are available for Python, which makes it easy to perform many tasks with just a few lines of code.
One such library is curl
, which allows Python developers to make HTTP requests to a server and receive responses.
This can be useful for various purposes, such as fetching data from an API, testing a website, or automating tasks involving interacting with a server.
In this article, we'll explore how to use curl
in Python and some of the common tasks that it can be used for.
Installing the curl library
Before we can use curl
in Python, we need to install it. The easiest way to do this is using pip
, the Python package manager. Simply open a terminal or command prompt and run the following command:
pip install pycurl
This will install the pycurl
library, which provides Python bindings for the curl
command-line tool.
Making HTTP requests with curl
Once curl
is installed, we can start making HTTP requests in Python. The pycurl
library provides a Curl
class that we can use to make requests. Here's an example of how to use it to make a simple GET request to a website:
import pycurl
c = pycurl.Curl()
c.setopt(c.URL, 'https://www.example.com/')
c.perform()
This code creates a Curl
object, sets the URL that we want to make the request to, and then uses the perform
method to send the request. This will make a GET request to the specified URL and return the response from the server.
We can also make other types of HTTP requests, such as POST, PUT, and DELETE, using the Curl
class. For example, here's how we would make a POST request with some data:
import pycurl
c = pycurl.Curl()
c.setopt(c.URL, 'https://www.example.com/')
c.setopt(c.POSTFIELDS, 'name=value')
c.perform()
This code is similar to the previous example, but it sets the POSTFIELDS
option to specify the data that we want to send with the request. This data will be sent in the body of the request as form data.
Handling responses
When we make a request with curl
, we usually want to do something with the response that we receive from the server. The Curl
class provides several methods that we can use to access the response data.
For example, we can use the getinfo
method to get information about the response, such as the HTTP status code and the size of the response body. Here's an example:
import pycurl
c = pycurl.Curl()
c.setopt(c.URL, 'https://www.example.com/')
c.perform()
print(c.getinfo(c.RESPONSE_CODE))
print(c.getinfo(c.SIZE_DOWNLOAD))
Common Questions
Can I use curl
with Python 3?
Yes, curl
is compatible with Python 3. In fact, the pycurl
library provides Python 3 bindings for curl
, so you can use it with Python 3 without any issues.
Can I use curl
to make requests to a local server?
Yes, curl
can be used to make requests to any server, including a local server that is running on your machine. Simply specify the URL of the local server in the setopt
method when you create the Curl
object.
Can I use curl
to upload files?
Yes, you can use curl
to upload files to a server. Simply use the setopt
method to specify the path to the file that you want to upload, and then use the perform
method to send the request.
Can I use curl
to set request headers?
Yes, you can use curl
to set request headers. The Curl
class provides a setopt
method that allows you to specify the headers that you want to include in the request. Here's an example:
import pycurl
c = pycurl.Curl()
c.setopt(c.URL, 'https://www.example.com/')
c.setopt(c.HTTPHEADER, ['Content-Type: application/json'])
c.perform()
In this example, we're setting the Content-Type
header to application/json
, which tells the server that the request body contains JSON data.
Can I use curl
to handle HTTPS requests?
Yes, curl
can be used to make HTTPS requests. Simply specify the URL of the HTTPS server in the setopt
method when you create the Curl
object. curl
will automatically handle the SSL/TLS negotiation and provide secure communication with the server.
In summary, curl
is a powerful library that allows Python developers to make HTTP requests and handle responses easily. It is widely used for a variety of purposes, including web development, data analysis, and automation. By using curl
, you can quickly and easily perform tasks that involve interacting with a server in your Python applications.