Handle Guzzle exception and get HTTP body?
Learn the simple steps to manage Guzzle exceptions and access the HTTP body from your requests. This guide makes handling errors and integrating APIs like Google News or Google Trends API straightforward and effective for your projects.

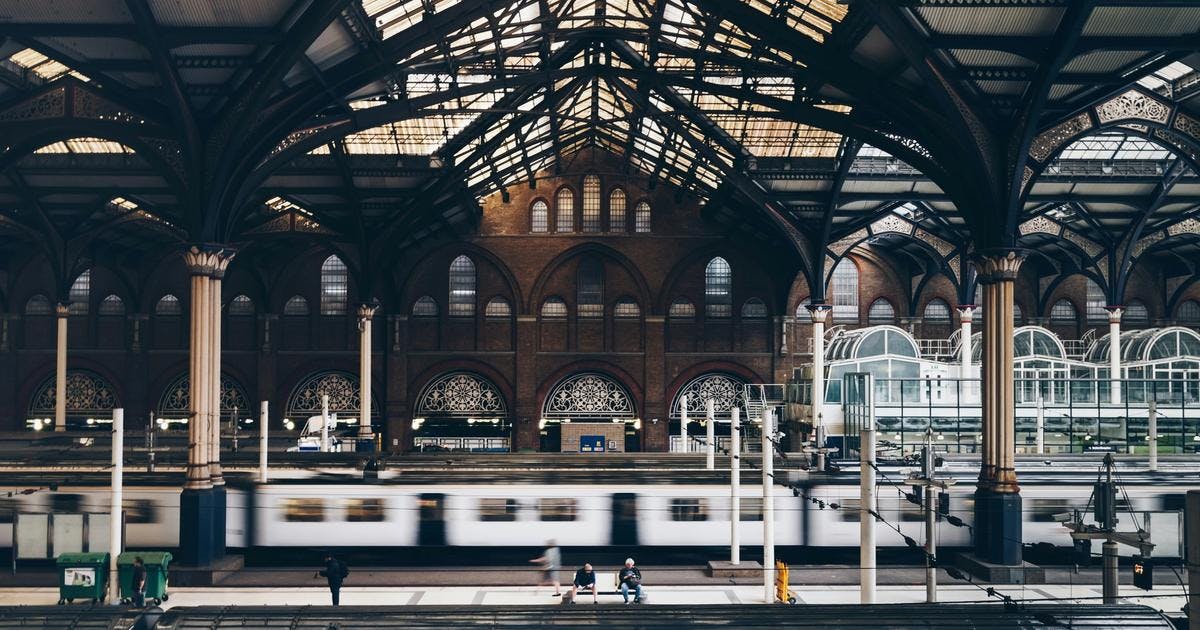
- Understanding Guzzle's Error Management
- How to Handle RequestException in Guzzle
- Catching Specific Errors
- Want to Learn More?
When working with web requests in your projects, encountering errors or exceptions can be common. If you're using Guzzle, a well-liked HTTP client for PHP, knowing how to manage these exceptions effectively is key. This easy-to-follow guide will show you how to handle Guzzle exceptions and retrieve the HTTP body from responses, ensuring your projects run smoothly. This is particularly handy when working with various APIs, such as integrating with the Google News API to fetch the latest news for your application.
Understanding Guzzle's Error Management
Guzzle structures its errors in a hierarchical way, meaning it organizes different types of errors under various categories. At the basic level, you might come across a RuntimeException
. But more specifically, when dealing with HTTP requests, the RequestException
is what you often need to deal with. This includes issues like bad server responses or too many redirects.
How to Handle RequestException
in Guzzle
If you're fetching data from a website and something goes wrong, Guzzle allows you to catch these errors neatly. Here's a simple example:
use GuzzleHttp\Client;
use GuzzleHttp\Exception\RequestException;
$client = new Client();
try {
$response = $client->get('https://example.com/api');
$body = $response->getBody(); // Here's where you get the content you wanted
} catch (RequestException $e) {
if ($e->hasResponse()) {
$response = $e->getResponse();
$body = $response->getBody(); // Even in errors, you can still look at the details
} else {
// Here's where you figure out what to do if there's a bigger problem, like a network issue
}
}
This approach not only helps in successful data fetching but also in scenarios where your application needs to interact with other services, for example, pulling data using the Google Trends API to analyze current trends.
Catching Specific Errors
For those who like to be more precise, Guzzle also has specific error classes for client errors (400s), server errors (500s), and other bad responses. By using these, you can fine-tune your error handling even more.
Want to Learn More?
Diving deeper into Guzzle and how to manage its exceptions will make your applications more robust. The official Guzzle documentation is packed with info to help you do just that.
Mastering how to catch and manage errors in Guzzle, and retrieve HTTP body content even when things go awry, can significantly improve your project's reliability. With this straightforward guide, you're now better equipped to handle whatever web requests throw at you, whether it's enhancing your app's functionality with the Google Images API or integrating other powerful tools from the web.