How do I do HTTP basic authentication with Guzzle?
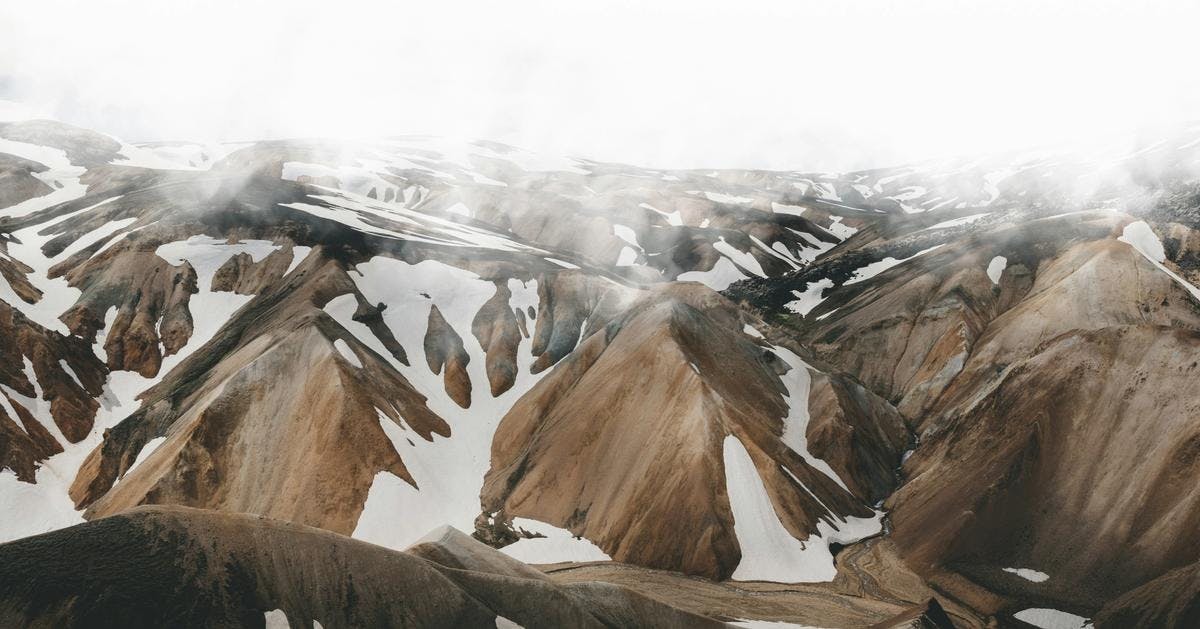
- Setting Global Authentication
- Authentication for Specific Requests
- Persisting Sessions Across Requests
- Exploring More with Guzzle
Implementing HTTP basic authentication securely with Guzzle is straightforward. This popular HTTP client for PHP facilitates secure access to APIs, making it an essential tool for projects that integrate with various online services. By setting up HTTP basic authentication, your application can safely interact with endpoints that require credentials.
Setting Global Authentication
Global authentication in Guzzle ensures that your credentials are automatically included with all requests made by the client. This approach is beneficial for projects with consistent authentication across all API requests, such as when fetching data from the Google Scholar API:
use GuzzleHttp\Client;
$client = new Client([
'auth' => ['username', 'password']
]);
$response = $client->get('https://httpbin.org/basic-auth/username/password');
$body = $response->getBody();
echo $response->getStatusCode() . PHP_EOL;
echo $body;
// Expected output shows successful authentication:
// 200
// {
// "authenticated": true,
// "user": "username"
// }
This setup is perfect for ensuring seamless access to protected resources.
Authentication for Specific Requests
For scenarios requiring different credentials across various endpoints, Guzzle provides flexibility to specify authentication details with each request:
$client = new GuzzleHttp\Client();
$response = $client->request('GET', 'https://httpbin.org/basic-auth/username2/password2', [
'auth' => ['username2', 'password2']
]);
$body = $response->getBody();
echo $response->getStatusCode() . PHP_EOL;
echo $body;
// Output confirms successful authentication:
// 200
// {
// "authenticated": true,
// "user": "username2"
// }
This method is invaluable for projects that interact with multiple APIs requiring unique credentials for each.
Persisting Sessions Across Requests
Guzzle's cookie handling feature enables seamless session management across requests, vital for web services tracking session states or personalization. This feature ensures your application maintains continuity without manual cookie management.
Exploring More with Guzzle
Guzzle's vast array of features extends well beyond authentication. For developers looking to deepen their understanding of Guzzle and explore its advanced capabilities, the official Guzzle documentation is an excellent resource.
By leveraging Guzzle for HTTP basic authentication, you can ensure secure and efficient interactions with protected APIs, enhancing your application's functionality and security stance.