How do you handle client error in Guzzle?

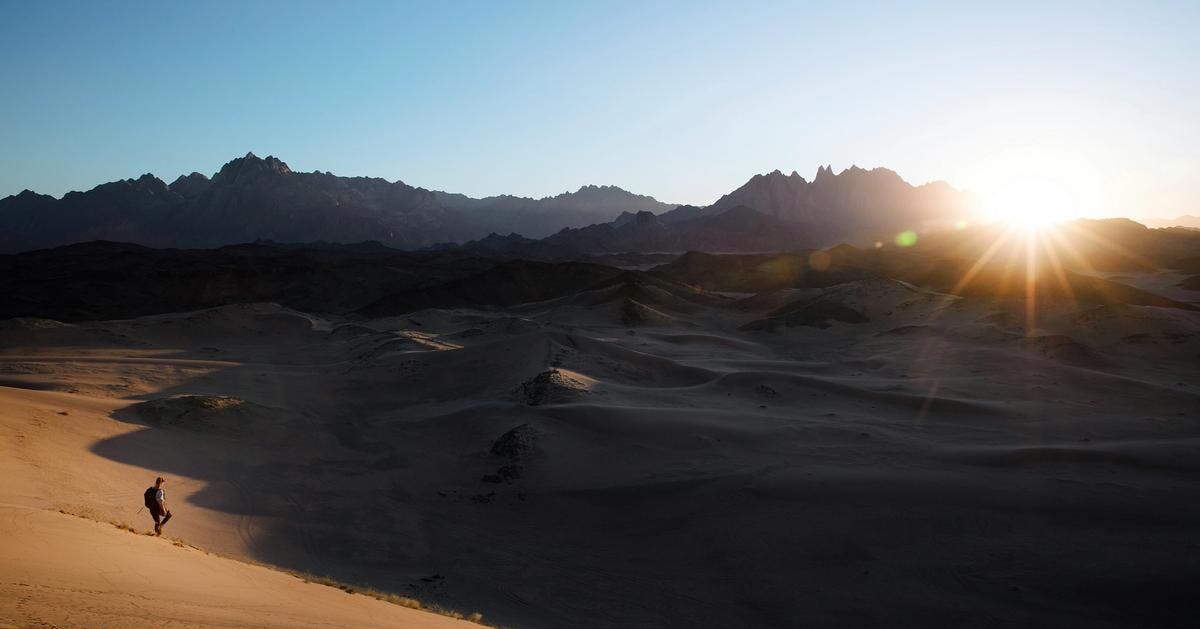
- What Are Client Errors?
- Catching Exceptions in Guzzle
- Example of Handling a 404 Not Found Error
- Tips for Better Error Management
- Conclusion
Handling client errors while using APIs in Guzzle is essential for every developer. This straightforward guide will help you manage these errors effectively, ensuring your application interacts seamlessly with various APIs, such as Google Trends API or Google News API.
What Are Client Errors?
Client errors are those within the 4xx status code range, indicating an issue with the request you made. For example, a 404 Not Found error signifies that the resource you’re attempting to access doesn't exist.
Catching Exceptions in Guzzle
Guzzle gives you the ability to catch errors through exceptions. You mainly have two approaches:
- Using RequestException: This is a broader method that can catch most general exceptions.
- Using ClientException: A more specific approach that catches client-related errors, such as 4xx status codes.
Example of Handling a 404 Not Found Error
Here’s a quick way to manage a 404 Not Found error:
use GuzzleHttp\Client;
use GuzzleHttp\Exception\ClientException;
$client = new Client();
try {
$response = $client->get('https://httpbin.org/status/404');
// Process the response here if no error occurs…
} catch (ClientException $e) {
// This is where you handle the error
$response = $e->getResponse();
$responseBody = $response->getBody()->getContents();
echo $response->getStatusCode() . PHP_EOL;
echo $responseBody;
}
// This will output:
// 404
This code snippet demonstrates that if a 404 error is encountered, Guzzle allows you to catch and handle it effectively, providing the error code and message.
Tips for Better Error Management
- Log errors: Keeping a log of errors can help identify and correct issues more quickly.
- Provide clear user feedback: When an error occurs, offer clear guidance to your users about what went wrong.
- Dive deeper with Guzzle docs: For more in-depth knowledge on error handling, consider the Guzzle documentation as an invaluable resource. It details Guzzle’s exception mechanism thoroughly.
Conclusion
Mastering client error handling in Guzzle is crucial for building robust applications that smoothly integrate with APIs like the Google Scholar API or the Google Images API. Through effective error management, your application can ensure reliable API communication and enhance overall user experience. Remember, efficiently handling errors is about preparedness and the right response to unforeseen issues.
Play