Nodejs Fetch API
Discover the capabilities and limitations of the Fetch API, which is now built into Node.js version 18.0.0 or later

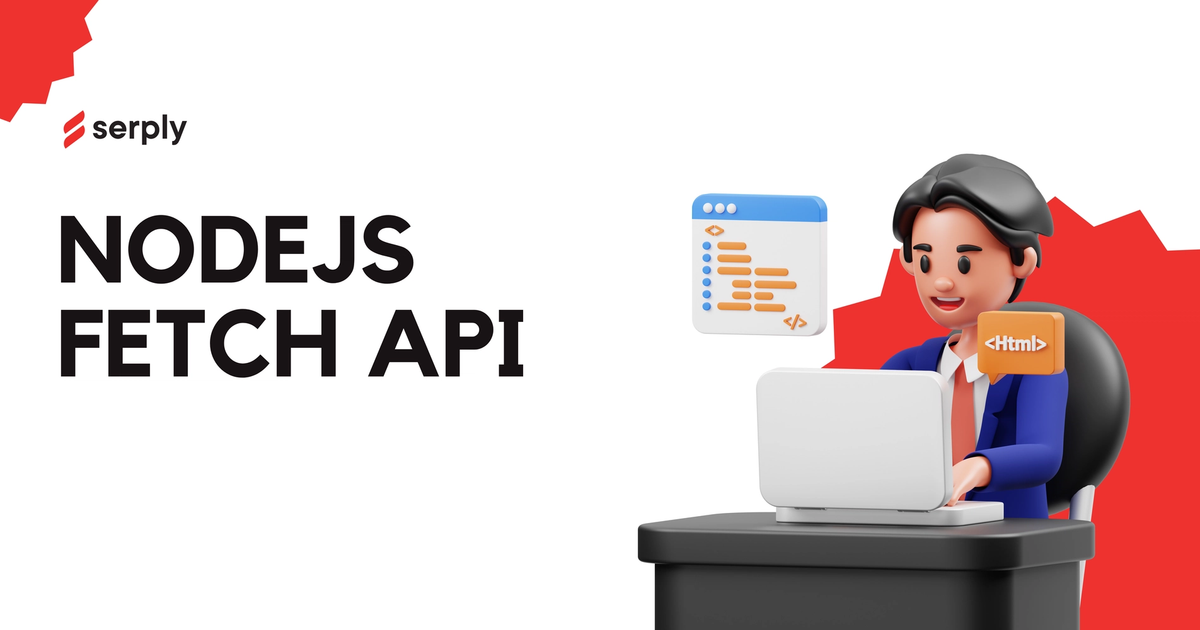
- The History of Web Requests
- Introducing the Fetch API
- Undici
- The Fetch API finds stability in Node.js v18
- Enhanced stability
- Improved compatibility
- Performance optimizations
- Benefits of using the Fetch API in Node.js
- No extra fetch package
- Cross-platform familiarity
- Faster implementation
- Code maintainability
- Drawbacks
- Lack of built-in progress events
- Limited support for older Node.js versions
- Complexity in handling cookies
- How to use the Fetch API
- Migrating to the official, stable Fetch
- Update Node.js version
- Remove dependency on external libraries
- Update code to use native Fetch
- Adjust options and headers
- Testing and debugging
- Conclusion
The Node.js community has been eagerly awaiting the stabilization of the Fetch API, a significant update that has finally arrived. This improvement is essential for developers as it introduces a uniform and contemporary method for making HTTP requests, applicable across both browser and server settings. To understand the importance of this development, exploring sources like the Google Trends API can provide insights into the growing interest in Node.js and related technologies over time.
The History of Web Requests
Back in the early stages of the internet, making asynchronous requests between different websites was a challenging task. Developers were forced to rely on awkward methods for cross-network interaction.
This changed in 1998 when Internet Explorer 5 introduced the XMLHttpRequest API. Originally, the XMLHttpRequest was created to retrieve XML data over HTTP, which is reflected in its name. However, it wasn’t long before the API began supporting other data types, including JSON, HTML, and plaintext.
While XMLHttpRequest was a groundbreaking solution at the time, the complexity of working with it increased as the web evolved. This complexity led to JavaScript frameworks, such as jQuery, creating abstractions that simplified the implementation process and made handling success and error responses more manageable.
Introducing the Fetch API
Launched in 2015, the Fetch API arrived as a modern alternative to the older XMLHttpRequest, quickly becoming the preferred method for making asynchronous web requests. Its main advantage over its predecessor is the use of promises, which offer a more straightforward and cleaner approach to asynchronous programming, steering clear of the notorious callback hell.
Although the Fetch API has been a part of web development for some time, its integration into Node.js’s core was delayed due to certain challenges. A key issue, as explained by a Node.js core contributor, was the Fetch API’s reliance on browser- specific features like the Web Streams API and the AbortController interface, which are used for aborting fetch requests. These components were not initially available in Node.js, complicating the process of incorporating Fetch into the Node.js environment.
Before Fetch’s incorporation into Node.js, the ‘request’ module stood as the go-to solution for HTTP requests within the Node ecosystem. However, the rapid evolution of the JavaScript landscape, marked by the adoption of new patterns such as async/await, gradually rendered the ‘request’ module outdated. The module did not support these new patterns, leading to its eventual deprecation. This shift underscores the JavaScript community’s move towards more modern and efficient programming practices.
Undici
In 2018, the Node.js ecosystem welcomed Undici, a new and improved HTTP/1.1 client that boasted features like pipelining and connection pooling. The Node core team dedicated significant effort to refining Undici, enhancing its performance, making sure its stability, and making sure it aligned with the broader goals of the Node project.
Undici played a pivotal role in the development of Node.js own fetch() function, offering a high-performance, standards-compliant way to make HTTP requests. By incorporating Undici directly into Node’s core, the developers provided the community with an efficient and speedy HTTP client. This groundwork was crucial for the introduction of a reliable Fetch API in Node.js version 18 and its versions, marking a significant step forward in Node.js’s capabilities for web development.
The Fetch API finds stability in Node.js v18
The launch of the Fetch API in Node.js version 18 is a significant step in the development of the Node.js ecosystem, providing developers with a consistent and reliable way to make HTTP requests. This much-anticipated release brings added features and advantages to the Fetch API, solidifying its status as a dependable method for HTTP requests in Node.js environments.
Enhanced stability
The key highlight of Node.js version 18 is the stabilization of the Fetch API. This development allows developers to confidently use this API, thanks to extensive testing and refinement that ensures its stability and reliability across different situations.
Improved compatibility
The stable release of Node.js version 18 addresses compatibility issues that might have appeared in earlier versions. With a focus on smooth integration, the Fetch API ensures a uniform experience in various environments. This makes it an adaptable tool for developers who are building applications that run on both the client and server sides.
Performance optimizations
In Node.js version 18, the Fetch API is optimized for speed, enhancing the efficiency of HTTP requests. This optimization leads to quicker response times and improved performance for applications, crucial for those managing a large number of simultaneous requests.
Benefits of using the Fetch API in Node.js
The integration of the Fetch API as a built-in module in Node.js is highly advantageous for the developer community. The benefits of this integration include:
No extra fetch package
The introduction of Fetch as native feature in Node.js could signal a shift away from third-party packages like node-fetch, got, and cross-fetch, which were developed for similar purposes. This change eliminates the need for an npm install command before executing network operations in Node.
Moreover, node-fetch, which has been the go-to fetch package for Node.js, transitioned to an exclusively ESM (ECMAScript Module) package. As a result, it can no longer be imported using Node’s require() function. With the native Fetch API, HTTP requests in Node environments are set to become more streamlined and intuitive.
Cross-platform familiarity
Developers who are already familiar with using the Fetch API in frontend development will find the transition to using the built-in fetch() method in Node seamless. This approach is significantly more straightforward and intuitive than relying on external packages to accomplish similar tasks within a Node environment.
Faster implementation
The new Fetch implementation in Node.js is built on Undici, a fast, reliable and specification-compliant HTTP client. Consequently, you can expect enhanced performance from the Fetch API as well.
Code maintainability
The Fetch API being stable in Node.js version 18 makes it easier to keep code in good shape. When developers use a common method for making HTTP requests, managing and updating the code becomes simpler. This approach leads to more stable projects over time and reduces the chances of making mistakes.
Drawbacks
The browser’s Fetch API comes with its own set of limitations, and these are likely to carry over to the new Fetch implementation in Node.js:
Lack of built-in progress events
The fetch API in Node.js doesn’t include built-in features to track progress during file uploads or downloads. Developers looking for detailed monitoring of progress might find this lack of feature challenging and may have to explore other libraries or approaches to meet their needs.
Limited support for older Node.js versions
The Fetch API is stable in the latest Node.js versions, but projects on older versions might face compatibility issues. Developers in situations where updating Node.js isn’t an option might have to use different libraries or create their own solutions.
Complexity in handling cookies
The Fetch API manages cookies like a browser does, which can lead to surprises in a Node.js setting. Developers need to be careful and make sure they set things up correctly for their particular needs when working with cookies.
How to use the Fetch API
The Fetch API is a high-level function that, at its simplest, accepts a URL and returns a promise. This promise resolves with the response from the requested URL.
fetch("https://jsonplaceholder.typicode.com/todos/1")
.then((response) => {
// Do something with response
})
.catch(function (err) {
console.log("Unable to fetch -", err);
});
The Fetch API is a high-level function that, at its simplest, accepts a URL and returns a promise. This promise resolves with the response from the requested URL.
Migrating to the official, stable Fetch
Switching to the stable Fetch API in node.js means you’ll need to update your existing code to use the built-in Fetch functionality. Here’s how to go about migrating your code:
Update Node.js version
Make sure your Node.js is version 18 or newer. To see what version you have now, use this command:
node -v
If your Node.js isn’t at least version 18, you need to update it. You can either use a tool like nvm(Node Version Manager) or get the latest version straight from the official Node.js website.
Remove dependency on external libraries
If you’ve been using an external library like node-fetch or any other custom HTTP library for requests in Node.js, you can now remove those dependencies. The stable Fetch API is available natively, making external libraries unnecessary for this purpose:
npm uninstall node-fetch
Update code to use native Fetch
Switch out any code that uses an external library for making requests with the native fetch implementation. Make sure to update the syntax and manage promises properly.
const fetch = require('node-fetch');
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
After:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Adjust options and headers
Check and modify any options or headers in your requests as needed. The native Fetch API might work differently or offer additional features. Look at the official documentation for any particular requirements.
Testing and debugging
Once you’ve made these updates, carefully test your application to ensure the switch to the native Fetch API hasn’t caused any issues. Pay close attention to how errors are handled, how timeouts are set, and any specific logic tied to HTTP requests.
Conclusion
The node.js Fetch API becoming stable is a significant achievement, thanks to the hard work of the Node.js core team and the contribution of the high-performance HTTP client library, Undici. This development is a major advancement for developers.
With this stable version, developers gain various advantages, such as making HTTP requests in both browser and server settings. Thanks to the Fetch API’s simplicity, flexibility, and speed enhancements, developers can now have a more streamlined and uniform experience.