How to Download an Image with Python
One common task that Python is often used for is downloading files from the internet, such as images. This article will explore how to download an image with Python.

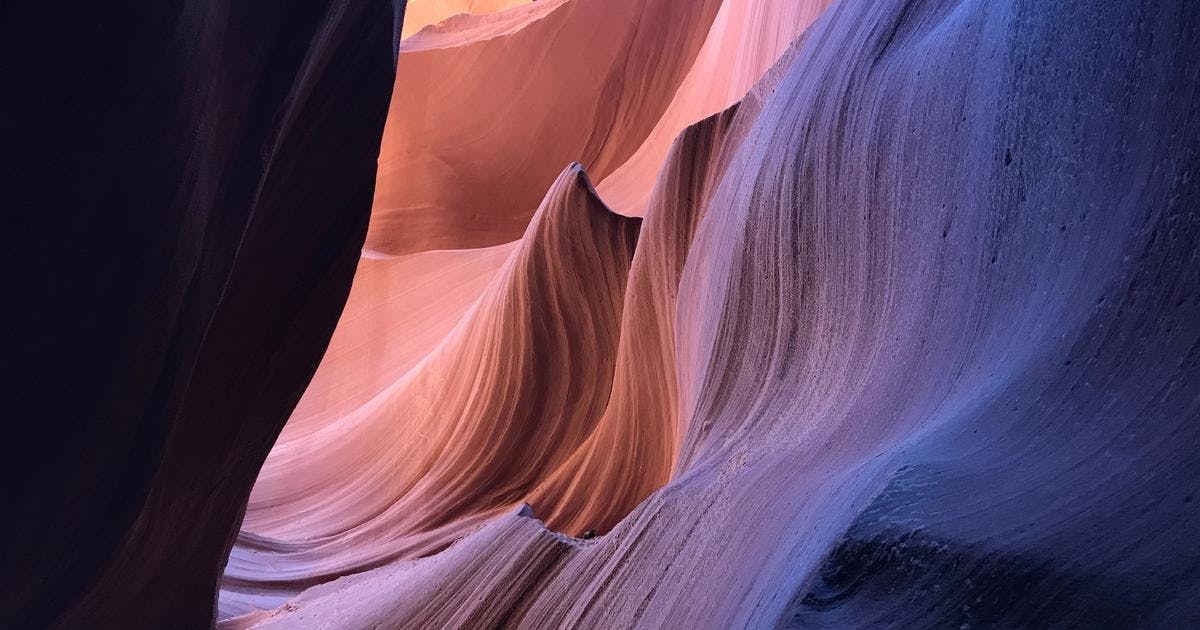
Python is a popular programming language that is widely used for various purposes, including web development, data analysis, and automation.
One common task that Python is often used for is downloading files from the internet, such as images.
This article will explore how to download an image with Python.
Using the requests library
The easiest way to download an image with Python is by using the requests
library. This library provides a simple interface for making HTTP requests. To use requests
, you need to install it first. You can do this using pip
, the Python package manager, like this:
pip install requests
Once requests
is installed, you can start using it to download an image.
Here's an example:
import requests
url = 'https://www.example.com/image.jpg'
response = requests.get(url)
with open('image.jpg', 'wb') as f:
f.write(response.content)
This code uses the requests.get
method to make a GET request to the specified URL. This sends a request to the server and receives a response containing the image data. The code then saves the image data to a local file.
Handling errors
When downloading files with Python, it's essential to handle errors that might occur. For example, the server might return an error if the URL is invalid, or if the image does not exist. To handle errors, we can use the try
and except
statements in Python. Here's an example:
import requests
url = 'https://www.example.com/image.jpg'
try:
response = requests.get(url)
response.raise_for_status()
except requests.exceptions.HTTPError as e:
print(e)
else:
with open('image.jpg', 'wb') as f:
f.write(response.content)
In this code, we're using the try
statement to try to request the specified URL. If an error occurs, the except
statement will catch it and print the error message. If the request is successful, the code in the else
block will be executed, which saves the image to a local file.
Conclusion
In this article, we've seen how to download an image with Python using the requests
library. We've looked at making a GET request to a URL and saving the image data to a local file.
We've also seen how to handle errors that might occur during the download process. By using the techniques described in this article, you can easily download images with Python in your projects.
FAQ
Can I use Python to download other types of files?
Yes, you can use Python to download any file from the internet. The requests
library is versatile and can request any URL, not just image URLs.
Can I download multiple images at once with Python?
Yes, you can use Python to download multiple images at once. You can use a loop to make multiple requests and save the images to separate files. Here's an example:
import requests
urls = ['https://www.example.com/image1.jpg', 'https://www.example.com/image2.jpg', 'https...]