Scrape Bing Search Results
This article will show you the step-by-step process of scraping Bing, including everything from setting up your scraping tool to executing the scrape and storing the data.

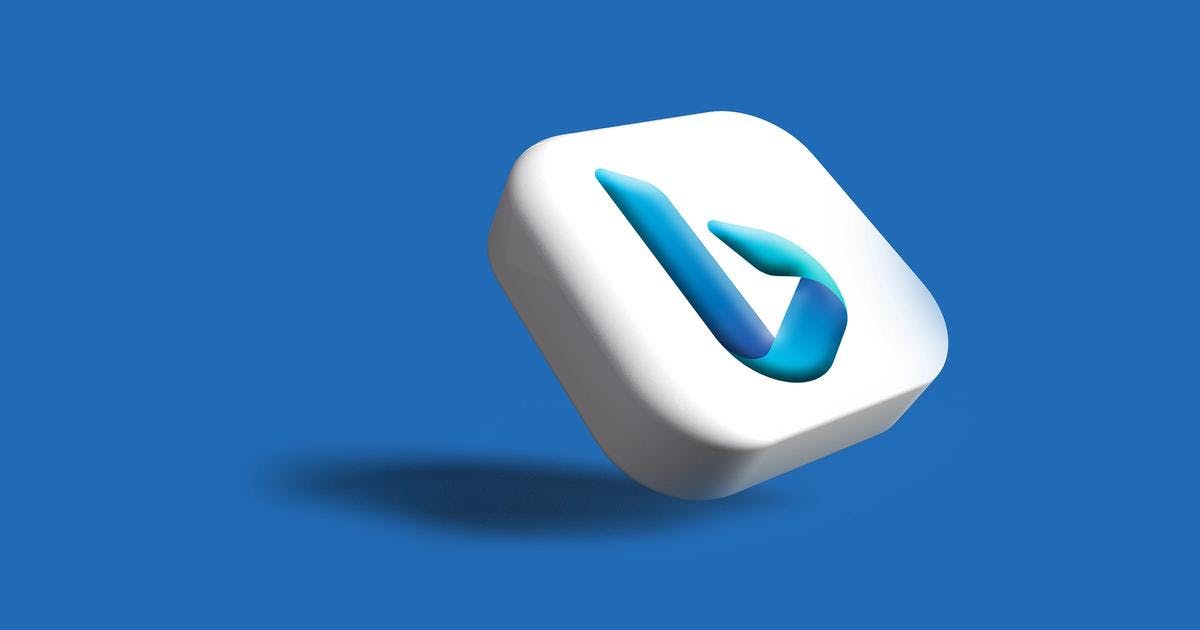
- Setting Up Your Scraping Tool
- Understanding the Structure of Bing
- Creating Your Scraping Spider
- Running Your Scraping Spider
- Storing the Data
Setting Up Your Scraping Tool
The first step in scraping Bing is to choose the right scraping tool. Many tools are available, each with its own features and capabilities.
Some popular scraping tools include Scrapy, BeautifulSoup, and Selenium.
In this guide, we'll use Scrapy, one of the most popular and powerful scraping tools available.
Once you've chosen your scraping tool, you'll need to install it. Installing Scrapy is straightforward and can be done using the command line.
Understanding the Structure of Bing
Before you start scraping Bing, it's essential to understand the website's structure. This will allow you to identify the data you need to scrape and to extract it properly.
Bing is structured similarly to most search engines, with a search bar at the top of the page and a list of results below. The results are displayed in a grid format, with each result containing a title, a description, and a link.
Creating Your Scraping Spider
Once you've set up your scraping tool and understand the structure of Bing, it's time to create your scraping spider. A scraping spider is a program that performs the actual scrape.
In Scrapy, a spider is created using a Python script. The script defines the structure of the scrape and the data to be extracted.
Here's an example of a simple Scrapy spider that scrapes the title and description of results from a Bing search:
import scrapy
class BingSpider(scrapy.Spider):
name = "bing"
start_urls = [
'https://www.bing.com/search?q=scraping+bing',
]
def parse(self, response):
for result in response.css('li.b_algo'):
yield {
'title': result.css('h2 a::text').get(),
'description': result.css('p::text').get(),
}
Running Your Scraping Spider
Once your spider is set up, you're ready to run it. To run the spider, open the command line and navigate to the directory where your spider is located. Then run the following command:
scrapy crawl bing
The scrape will start, and the data will be extracted and displayed in the command line.
Storing the Data
Finally, you'll need to store the data that you've scraped. This can be done in various ways, including storing it in a database, writing it to a file, or sending it to an API.
In this guide, we'll be storing the data in a CSV file. To do this, add the following code to your spider:
import csv
class BingSpider(scrapy.Spider):
...
def __init__(self):
self.csv_file = open('bing.csv', 'w')
self.csv_writer = csv.writer(self.csv_file)
self.csv