What is XPath in Selenium?
XPath is a language that is used for navigating through an XML document, such as an HTML page. In the context of Selenium, XPath can be used to locate elements on a web page, enabling you to find the elements you want to interact with and perform actions on them. In this article, we cover how to use xpath in Selenium.

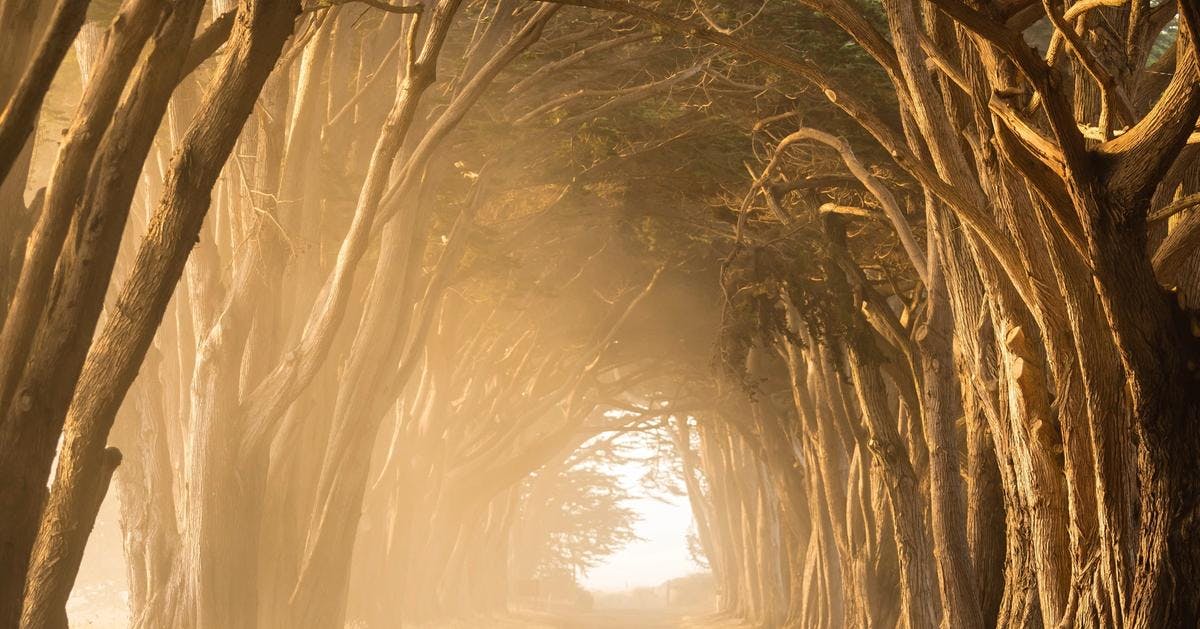
Selenium is a popular tool for automating web browsers. It allows you to write scripts that can interact with a website, simulating a user's actions such as clicking buttons, entering text, and navigating pages.
One of the key features of Selenium is its ability to find elements on a web page using various techniques, such as CSS selectors and XPath expressions.
This article will focus on XPath, a language for selecting elements in an XML or HTML document.
What is XPath?
XPath is a language for selecting elements in an XML or HTML document. It uses a path-like syntax to identify elements based on their attributes, such as their tag name, ID, and class.
For example, consider the following HTML code:
<html>
<body>
<h1>Hello, world!</h1>
<p>This is a paragraph.</p>
</body>
</html>
To select the h1
element using XPath, we could use the following expression:
//h1
This expression uses the //
operator to search for the h1
element anywhere in the document. It is similar to using the /
operator to search for an element in a file system, where the /
character separates the different parts of the path.
In XPath, we can also use the @
symbol to specify an attribute of an element. For example, selecting the p
element with the class
attribute set to main
, we could use the following expression:
//p[@class='main']
This expression uses the []
syntax to specify a condition that the element must satisfy to be selected. In this case, the condition is that the class
attribute of the p
element must be equal to main
.
Using XPath in Selenium
To use XPath in Selenium, you will first need to have Selenium installed on your system. You can do this by using a package manager like pip to install the Selenium Python package. Once you have Selenium installed, you can use the find_element_by_xpath
method to search for elements on a web page using an XPath query.
Selenium provides several methods that you can use to find elements on a web page using XPath expressions.
- Locate an element by its ID:
//element[@id='ID_VALUE']
- Locate an element by its class:
//element[@class='CLASS_VALUE']
- Locate an element by its name:
//element[@name='NAME_VALUE']
- Locate an element by its attribute value:
//element[@attribute='ATTRIBUTE_VALUE']
- Locate an element by its text content:
//element[text()='TEXT_CONTENT']
These are just a few examples of how you can use XPath to locate elements on a web page. XPath is a powerful language that allows you to create complex queries to find the elements you want, based on a wide variety of attributes and criteria. You can use XPath in Selenium to find elements on a page and interact with them in a variety of ways, such as clicking on a button, filling out a form, or extracting information from a page.
Once you have located the element you want using XPath, you can interact with it in a variety of ways. For example, you can use the click
method to click on a button, the send_keys
method to enter text into a form, or the text
attribute to extract the text content of an element.
Here is an example of how to use XPath in Selenium to locate and interact with an element:
from selenium import webdriver
# Create a new Chrome webdriver
driver = webdriver.Chrome()
# Navigate to a web page
driver.get("https://www.example.com")
# Use XPath to locate an element on the page
element = driver.find_element_by_xpath("//a[@id='example-link']")
# Interact with the element
element.click()
XPath is a powerful language that allows you to create complex queries to find the elements you want on a web page. However, it can be challenging to learn and use, especially for those who are new to Selenium and web development. If you are having trouble using XPath in Selenium, there are many resources available online, such as tutorials and documentation, that can help you to learn and understand how to use XPath effectively.
Frequently asked questions:
Is XPath the only way to locate elements in Selenium?
No, XPath is not the only way to locate elements in Selenium. There are other methods and techniques that you can use, such as using CSS selectors or locating elements by their ID, class, or name. The method that you use will depend on your specific requirements and the structure of the web page you are working with.
Can XPath be used with other web browsers besides Chrome?
Yes, XPath can be used with other web browsers besides Chrome. Selenium supports a wide range of web browsers, including Firefox, Safari, and Edge, and you can use XPath with any of these browsers to locate elements on a web page.
Are there any limitations to using XPath in Selenium?
There are some limitations to using XPath in Selenium, such as the complexity of the XPath queries you can use and the performance of the queries on large web pages.