Google SERP Scraper with Python: Simple Search Insights
Discover how to create a simple Google SERP scraper using Python to gain valuable search insights. This guide covers everything from setup to data analysis, along with legal considerations and best practices.

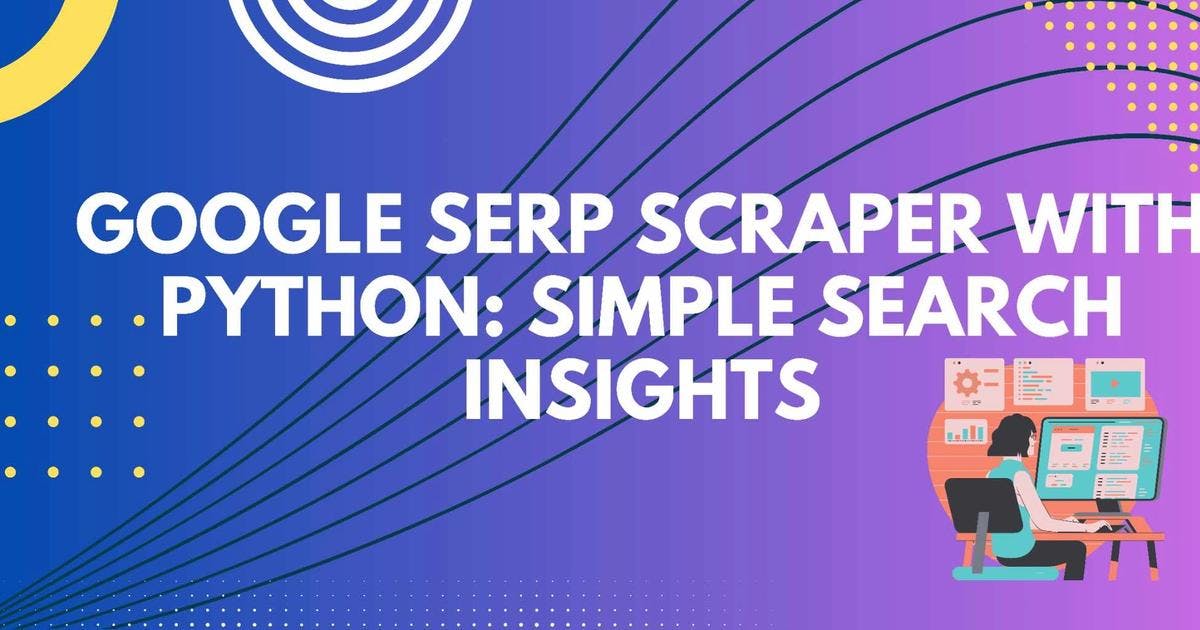
- Introduction to Google SERP Scraping
- The Basics of Python for Web Scraping
- Setting Up Your Python Environment
- Creating a Simple Google SERP Scraper
- Understanding the Legalities of Web Scraping
- Extracting Key SERP Data with Python
- Handling Pagination in SERP Scraping
- Storing and Analyzing Scraped Data
- Optimizing the Python SERP Scraper for Efficiency
- Common Challenges and Solutions in SERP Scraping
- Integrating SERP Data into SEO Strategies
- Advanced SERP Scraping Techniques
- SERP Scraping Best Practices
- Future Trends in SERP Scraping
- Conclusion
In the realm of SEO and digital marketing, understanding Google's Search Engine Results Pages (SERP) is crucial. With Python, creating a SERP scraper can provide valuable insights into search trends, keyword rankings, and competitive analysis. This guide walks you through building a basic Google SERP scraper with Python.
Introduction to Google SERP Scraping
Search Engine Results Page (SERP) scraping is the process of programmatically gathering data from search engine results, a practice that has become increasingly vital in the realms of SEO analysis, market research, and comprehending search engine behavior.
This technique allows for the extraction of various types of data from SERPs, including rankings, featured snippets, and organic and paid search results. SERP scraping is instrumental for SEO professionals and digital marketers as it provides insights into how search engines like Google rank websites, what kind of content is being prioritized, and how competitors are performing.
It's also crucial for market research, offering real-time data on market trends, customer preferences, and competitive landscapes. Understanding these aspects can help in refining SEO strategies, tailoring content to target audiences, and making informed decisions based on current market dynamics.
For an in-depth exploration of SERP scraping, Serply's blog on Google SERP scraping is a comprehensive resource. This blog delves into the nuances of SERP scraping, providing guidance on ethical scraping practices, navigating the technical challenges, and leveraging the extracted data for strategic advantages. Additionally, for those interested in the technical aspects and coding required for effective SERP scraping, Serply's Google Crawl API offers valuable insights and tools. By integrating SERP scraping into your digital strategy, you can gain a competitive edge in understanding and adapting to the ever-evolving digital landscape.
The Basics of Python for Web Scraping
Python, renowned for its simplicity and powerful library ecosystem, stands out as the programming language of choice for web scraping tasks. It offers a range of libraries specifically designed to ease the process of extracting and manipulating data from web pages.
Key among these libraries are Requests and BeautifulSoup. Requests is a Python HTTP library that allows you to send HTTP requests easily, enabling the retrieval of content from web pages. BeautifulSoup, on the other hand, is used for parsing HTML and XML documents, allowing for efficient data extraction from web page elements.
These libraries work in tandem to simplify the complexities of web scraping, making it accessible even to beginners. For those new to Python and web scraping, a great starting point is the official Python documentation, which provides comprehensive and beginner-friendly guides.
This documentation not only covers the fundamentals of Python programming but also offers insights into its various libraries and their applications in web scraping.
Additionally, for practical examples and advanced scraping techniques, exploring resources like Serply's blog on scraping with Python can be incredibly beneficial. This blog offers step-by-step tutorials and use cases, helping beginners and experienced programmers alike to harness the full potential of Python for web scraping tasks.
By mastering Python and its relevant libraries, you can efficiently gather and process web data, which is crucial for a variety of applications, including market research, SEO analysis, and data journalism.
Setting Up Your Python Environment
Before starting, ensure Python is installed on your system along with the necessary libraries. You can install libraries using pip:
pip install requests beautifulsoup4
Creating a Simple Google SERP Scraper
Here's a basic Python script to scrape Google search results:
import requests
from bs4 import BeautifulSoup
defgoogle_search(query):
url = f"https://www.google.com/search?q={query}"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
for result in soup.find_all('div', class_='r'):
title = result.find('h3').text
link = result.find('a')['href']
print(f"Title: {title}\nLink: {link}\n")
google_search("Python web scraping")
This script searches Google and prints out the titles and links of search results.
Understanding the Legalities of Web Scraping
Navigating the legal landscape of web scraping is crucial to ensure ethical and lawful data collection practices. While web scraping can be a powerful tool for gathering information from websites, it's essential to recognize and respect the legal boundaries that govern this practice. A key aspect of lawful web scraping is adhering to the guidelines set out in a website's robots.txt file. This file, typically located in the root directory of a website, specifies the protocols for automated access to the site, including which parts can be scraped and which should be left alone.
Additionally, it's important to consider the legality of scraping specific types of data, as certain information may be protected under copyright or privacy laws. For a comprehensive understanding of these legal considerations, Serply's legal documentation on web scraping offers valuable insights. This resource delves into the legal nuances of web scraping, providing guidance on how to conduct scraping activities without infringing on copyright or privacy rights. It also highlights the importance of being aware of and compliant with different countries' laws regarding data collection.
By staying informed about the legal aspects of web scraping and adhering to ethical practices, you can ensure that your data collection methods are not only effective but also legally sound.
Extracting Key SERP Data with Python
Python's versatility in web scraping extends to extracting various elements from Search Engine Results Pages (SERP). While basic scripts might focus on titles and links, Python allows you to refine and expand your scraping capabilities to include other critical data elements such as meta descriptions, featured snippets, and other SERP features.
By customizing your Python script, you can target specific data points relevant to your research or SEO analysis. This tailoring involves not only fetching the usual suspects like titles and URLs but also digging deeper to extract more nuanced data like ratings, reviews, or even the presence of rich snippets. The key is to identify the specific data you need and modify your script accordingly, utilizing Python libraries such as BeautifulSoup or Scrapy. These libraries offer powerful parsing capabilities, enabling you to navigate and extract from the complex structures of HTML or XML pages.
For guidance on customizing Python scripts for advanced SERP data extraction, resources likeSerply's Python tutorials can be particularly helpful, offering practical examples and tips.
Handling Pagination in SERP Scraping
When scraping data from SERPs, one crucial aspect to consider is pagination, as search results often span multiple pages. To effectively navigate through these pages, your Python script must be equipped to handle pagination. This typically involves modifying the query URL to include parameters for page numbers or offsets.
By incrementally adjusting these parameters, your script can systematically access and scrape data from subsequent SERP pages. Handling pagination is essential for comprehensive data collection, ensuring you gather a complete dataset that encompasses the breadth of search results for your query. The process requires a careful balance of efficiency and thoroughness, making sure that your script retrieves all relevant data without getting trapped in infinite loops or overloading the server.
For a deeper understanding of implementing pagination in Python scripts, Serply's documentation on SERP pagination provides valuable insights and best practices. This resource will guide you through the technical aspects of managing pagination in web scraping, ensuring efficient and effective data collection across multiple SERP pages.
Storing and Analyzing Scraped Data
Once you have successfully scraped data using Python, the next crucial steps are storing and analyzing this data effectively. For storage, options range from databases, such as MySQL or MongoDB, to simpler formats like CSV files, which can be easily managed through spreadsheet applications. Python's ecosystem offers robust libraries like pandas, which is particularly adept at handling and analyzing data in formats like CSV or Excel. Pandas provide functionalities for cleaning, transforming, and analyzing large datasets, enabling you to extract valuable insights from the scraped data.
This could involve anything from identifying trends and patterns to performing complex statistical analyses. By efficiently storing and analyzing your data, you transform raw scraped information into actionable intelligence, which can be instrumental in market research, SEO strategy formulation, or competitive analysis. To further enhance your data analysis skills using Python,Serply's tutorials on data analysis can be an invaluable resource, offering practical guidance and advanced techniques in data handling and interpretation.
Optimizing the Python SERP Scraper for Efficiency
In web scraping, especially when dealing with large volumes of data or frequent scraping activities, efficiency becomes paramount. Optimizing your Python SERP scraper involves several strategies to ensure it operates smoothly and effectively.
Firstly, managing request rates is critical to prevent overloading the server and getting your IP address blocked. Implementing caching mechanisms can significantly improve efficiency by storing previously scraped data, thus reducing redundant server requests.
Another advanced technique is the use of asynchronous programming, which allows your scraper to handle multiple tasks concurrently, speeding up the data collection process. Libraries like asyncio in Python can be utilized for this purpose, enabling you to perform concurrent HTTP requests, which is particularly beneficial when scraping data across multiple pages.
By focusing on these optimization techniques, you can enhance the performance and reliability of your Python SERP scraper, ensuring faster data collection while minimizing the risk of server issues or IP bans. For insights into efficient scraper design and best practices, exploring resources like Serply's blog on Python scripting can provide valuable tips and strategies to refine your scraping tool.
Common Challenges and Solutions in SERP Scraping
In the practice of SERP scraping, several common challenges often emerge, each requiring specific solutions to ensure efficient data collection. One significant challenge is handling dynamic JavaScript content, which is increasingly prevalent in modern web applications. Traditional scraping tools might struggle to render and scrape such content, as it is dynamically loaded.
To tackle this, one effective solution is the use of headless browsers, which can render JavaScript just like a standard browser but without the graphical interface. This can be achieved using Python libraries like Selenium, which automates web browser interaction, allowing your scraper to access and extract data from dynamically generated web pages.
Another frequent obstacle in SERP scraping is dealing with CAPTCHAs, designed to block automated access. Solutions to this challenge can range from implementing IP rotation to using anti-CAPTCHA services, which can bypass CAPTCHA checks, albeit raising ethical considerations.
For a deeper understanding of these challenges and solutions, exploring resources like Serply's documentation on web scraping challenges can provide comprehensive insights and strategies to effectively navigate these common obstacles in SERP scraping.
Integrating SERP Data into SEO Strategies
Leveraging the data obtained from SERP scraping can significantly enhance your SEO strategies. By analyzing the scraped data, you gain valuable insights into keyword rankings, competitor performance, and prevailing market trends. This information is crucial in refining your SEO efforts. For instance, understanding how your website and competitors rank for certain keywords can inform your content optimization and keyword targeting strategies.
Tracking changes in keyword rankings over time also helps in identifying emerging trends and adapting your SEO approach accordingly. Additionally, analyzing the types of content that rank highly in SERPs, such as video content or featured snippets, can guide you in diversifying and enriching your content strategy.
By effectively integrating this data into your SEO planning, you can make informed decisions, identify new opportunities, and stay ahead in the competitive landscape of search engine rankings. To maximize the benefits of your scraped SERP data in SEO, Serply's blog on integrating SERP data into SEO offers practical tips and insights on how to strategically use this data to enhance your website's search engine visibility and performance.
Advanced SERP Scraping Techniques
For those delving deeper into the field of SERP scraping, employing advanced techniques can significantly enhance the efficiency and effectiveness of data collection. One such advanced approach is the integration of machine learning for pattern recognition within SERP data.
This method allows for the automated identification of trends and anomalies in large datasets, providing deeper insights into search engine behavior and SEO dynamics. Another strategy for advanced users is deploying scrapers in the cloud. Cloud deployment offers increased performance and scalability, accommodating larger volumes of data and more complex scraping tasks without the limitations of local hardware resources. Utilizing cloud platforms also provides the flexibility to scale your scraping operations up or down based on your requirements.
For more sophisticated scraping techniques and insights, resources like Serply's blog on advanced SERP scraping can be invaluable, offering guidance on implementing these cutting-edge approaches in your scraping projects.
SERP Scraping Best Practices
Adhering to best practices in SERP scraping is fundamental to ensure ethical and sustainable data collection. This includes respecting the terms of service of websites, scraping data responsibly, and prioritizing data privacy. Ethical scraping practices not only prevent legal complications but also maintain the integrity of your scraping activities. Respecting robots.txt files, avoiding excessive server load, and anonymizing data to protect user privacy are key aspects of responsible scraping.
Additionally, staying informed about the legal landscape surrounding web scraping is crucial to avoid infringing on intellectual property rights or privacy laws. For comprehensive guidelines on ethical SERP scraping practices,Serply's legal documentation on web scraping offers essential insights, helping you navigate the complexities of legal and ethical considerations in web scraping.
Future Trends in SERP Scraping
Keeping up with the latest trends in SERP scraping is crucial in an ever-evolving digital landscape. One significant trend is the advancement of AI and machine learning in web scraping. These technologies are becoming increasingly adept at automating data extraction processes, recognizing complex patterns, and even making predictive analyses.
Additionally, the legal landscape surrounding web scraping is continuously evolving, with new regulations and guidelines emerging in response to technological advancements and privacy concerns. Staying updated with these developments is key to adapting your scraping strategies to the latest technologies and legal requirements.
To stay informed about the latest trends and advancements in SERP scraping, regularly visiting resources like Serply's blog on emerging trends in SEO is highly beneficial. This resource provides updates on new technologies, legal changes, and best practices in the field of SERP scraping, ensuring that your scraping techniques remain state-of-the-art and compliant with current standards.
Conclusion
In conclusion, leveraging a Python-based Google SERP scraper is a strategic move for anyone in digital marketing or SEO, providing essential insights into the search landscape. This tool enables you to gather and analyze data from search engine results, offering a deeper understanding of your website's performance, competitor strategies, and emerging market trends.
By adhering to the guidelines and best practices for ethical and efficient web scraping, such as respecting website protocols and utilizing Python's powerful libraries, you can effectively harness this technology. The insights gained from SERP scraping are invaluable in refining your digital marketing and SEO strategies, helping you to adapt to changing search algorithms and user behaviors.
Ultimately, a well-implemented SERP scraper is not just a data collection tool, but a means to enhance your online visibility and drive successful digital outcomes.